CoCreate Modeling code examples: Secure a part against sectioning in Annotation views
The task at hand
Sometimes you do not want a part to be sectioned in Annotation section views (or
docuplanes). In such a case, you can either use the built-in
functionality in Annotation to "secure" a part, or you can programmatically
assign a special "don't section" flag to a part.
The standard functionality in Annotation is usually preferrable because
it allows to precisely define in which views the part should be protected
against sectioning. However, the Annotation module must be activated to
use the standard functionality, but maybe you want to globally prevent
a part from being sectioned, and you want to do that at part creation time
(i.e. while using Modeling). Developer's Kit to the rescue: A global
"don't section" flag can be set using APIs from the Developer's
Kit which have become available with OSDM 2005. We will now use those
APIs to develop a simple dialog.
The dialog code
For the impatient, here is the complete dialog:
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Description: "Secures" a part against (2D and 3D) Annotation sections
;; Author: Claus Brod
;; Language: Lisp
;;
;; (C) Copyright 2005 Claus Brod, all rights reserved
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
(in-package :clausbrod.de)
(use-package :oli)
(sd-defdialog 'secure_part
:dialog-title "Secure part" :toolbox-button t
:variables
'(
(Part :value-type :part
:after-input
(sd-set-variable-status 'Secured
:value (sd-inq-secure-section-flag Part)))
(Secured :value-type :boolean
:initial-value (when Part (sd-inq-secure-section-flag Part))
)
:ok-action
'(sd-set-secure-section-flag Part :secure-section-flag Secured)
)
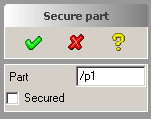
Among other things, the dialog code does the following:
- Defines a new command called
secure_part
which can be
run from the user input line and in other Lisp code.
- Creates a simple dialog (see image to the right) with the following
UI elements:
- OK button
- Cancel button
- Help button
- A part entry field consisting of a part button and a text field
- A flag entry field for the "secured" state consisting of
a checkbox and a label
- Registers an entry in the toolbox which starts the dialog
- When the dialog opens, the currently active part will
automatically be selected
- Allows to click the "Part" button and select any part
- When a part is selected, its current "secured" state is checked and
indicated in the "secured" checkbox
- Allows to change the "secured" state by clicking the checkbox
- Sets the new state of the part when clicking the OK button
- Allows to UNDO the change
- Registers the new command with the system so that it can be
used together with preselection (select a part, then
run the dialog, and the preselected part will appear in the
part entry field)
- When you run the command while the recorder is active, any
interactions with the dialog are automatically recorded
properly, just as if it was an internal command.
Quite a bit of functionality for just a few lines of very simple code!
The code explained
Let us now dissect the code step by step to learn how it works.
(in-package :clausbrod.de)
My own definitions in this file go to the Lisp package
:clausbrod.de
so that
if somebody else defines symbols with the same names, they won't collide.
(use-package :oli)
All the APIs from the Developer's Kit are defined in the
:oli
Lisp package,
and the above line makes them available for the following code.
(sd-defdialog 'secure_part
:dialog-title "Secure part" :toolbox-button t
Those two lines start the definition of an CoCreate Modeling
dialog. The dialog's name
is
secure_part
. A dialog always also defines a new command which can be used
from the user input line (example:
secure_part :part "/p1" :secured t
).
:dialog-title
defines what is displayed as the title of the dialog in the UI, and
the
:toolbox-button
keyword registers an entry in the toolbox from
which you can start the dialog.
The next two lines in the code start the
variables section:
:variables
'(
Variables are containers which can hold data of various useful types, such as:
- Numbers
- Directions
- Parts, assemblies, workplanes etc.
- Filenames
- Text
- Booleans (t/nil)
Some of these are simple data types (such as numbers or text fields), but some
are quite intelligent - for example, when you click a filename button, it will
automatically pop up a file browser and let you select a file; and when you
click a part button, it will automatically start CoCreate Modeling's selector with all its
advanced selection mechanisms.
In our case, we want to hold only two pieces of data - a part
and its "secured" status. The part is of type, well, "part", which is
indicated by the parameter for the
:value-type
keyword:
(Part :value-type :part
:after-input
(sd-set-variable-status 'Secured
:value (sd-inq-secure-section-flag Part)))
But what's that funny
:after-input
thing about? This is actually the most complicated
part of the whole dialog. Whenever a part is selected, the Lisp code which follows
:after-input
will automatically be executed. Let us rewrite this code for
slightly better readability:
(sd-set-variable-status 'Secured :value (sd-inq-secure-section-flag Part)))
That looks a little simpler already. So we use the Developer's Kit
function
sd-inq-secure-section-flag
to inquire the "secured" status of
Part
. This value is then transferred into the dialog variable
Secured
(which we will define in a second) using the
sd-set-variable-status
function.
(Secured :value-type :boolean
:initial-value (when part (sd-inq-secure-section-flag Part))
)
)
Aha, so here's the missing definition for the
Secured
variable. This variable is
of type
boolean
, so it can only hold a simple true/false decision - but that's
precisely what we need to indicate the current "secured" state of a part.
The variable
Part
is set to the current part when the dialog is started. But
what if no part has been set as the current part? The
:initial-value
check
for the
Secured
variable takes care of this situation.
To summarize: When we select a new part, we inquire its "secured" status
and copy that status into the
Secured
variable - which automatically
updates the UI!
Note the closing parenthesis after the definition for
Secured
- this closes
the list of variables which we started earlier.
Let us now finish up the dialog:
:ok-action
'(sd-set-secure-section-flag Part :secure-section-flag Secured)
)
The code which follows
:ok-action
is executed when the user clicks the OK
button. In our case, we want to run the
sd-set-secure-section-flag
function which changes the section flag for
Part
; here, we simply use
the current status of the
Secured
dialog variable (which the user can
change by clicking the checkbox) as the new "secured" status for the
selected part.
And that's all!
--
ClausBrod - 22 Mar 2005
--
DerWolfgang - 26 Apr 2005
:initial-value for Secured
Hallo Claus,
es fehlt eine Klammer im Code weiter oben
--
EdgarAufdermauer - 16 Nov 2007
to top