Yesterday, I introduced the problem of
how to automatically test Windows clipboard code in applications.
The idea is to move from manual and error-prone clickety-click style
testing to an automatic process which produces reliable results.
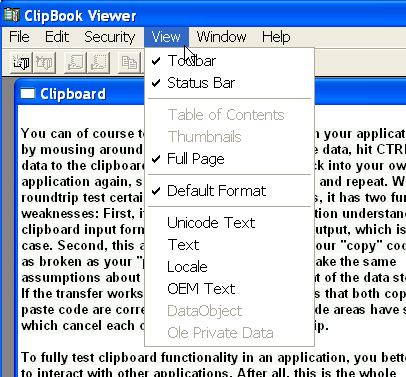
Unbeknownst to many, Windows ships with a fairly interesting tool
called the ClipBook Viewer (
clipbrd.exe
), which monitors
what the clipboard contains, and will even display the formats
it knows about.
This is quite helpful while developing and debugging clipboard code.
However, ClipBook Viewer can even help with test automation since it
can save the current clipboard contents to
*.CLP
files and load them
back into the clipboard later.
Which, in fact, is almost all we need to thoroughly and reliably
test clipboard code: We run some apps which produce
a good variety of clipboard formats which our own application needs
to deal with. We select some data, copy them to the clipboard, then
save the clipboard contents as a
*.CLP
file from ClipBook Viewer.
Once we have created a reasonably-sized clipboard file library,
we run ClipBook viewer and load each one of those clipboard
files in turn. After loading, we switch to our own app,
paste the data and check whether the incoming data makes sense to us.
Not bad at all!
But alas, I could not find a way to automate
the ClipBook Viewer via the command-line or COM interfaces. If someone
knows about such interfaces, I'm certainly most interested to hear about
them.
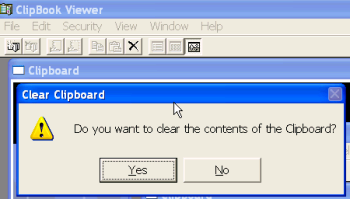
Luck would have it that only recently, I
blogged about poor man's automation via SendKeys. The idea is to write a small shell script
which runs the target application (here:
clipbrd.exe
), and then simulate how a user
presses keys to use the application.
clipbrd.exe
can be started with the name of a
*.CLP
file in its
command line, and will then automatically load this file. However,
before it pushes the contents of the file to clipboard, it will
ask the user for confirmation in a message box. Well, in fact, first
it will try to establish NetDDE connections, and will usually waste
quite a bit of time for this. The following script tries to take this
into account:
Set WshShell = WScript.CreateObject("WScript.Shell")
WshShell.Run("clipbrd.exe c:\temp\clip.CLP")
WScript.Sleep 5000 ' Wait for "Clear clipboard (yes/no)?" message box
WshShell.SendKeys "{ENTER}"
Now we could add some more scripting glue code to control our own
application, have it execute its "Paste" functionality and verify
that the data arrives in all its expected glory.
The above code is not quite that useful if we need to run
a set of tests in a loop; the following modified version is
better suited for that purpose. It assumes that all
*.CLP
files are stored in
c:\temp\clipfiles
.
Set WshShell = WScript.CreateObject("WScript.Shell")
WshShell.Run("clipbrd.exe")
WScript.Sleep 20000
startFolder="c:\temp\clipfiles"
set folder=CreateObject("Scripting.FileSystemObject").GetFolder(startFolder)
for each file in folder.files
WScript.Echo "Now testing " & file.Path
OpenClipFile(file.Path)
' Add here:
' - Activate application under test
' - Have it paste data from the clipboard
' - Verify that the data comes in as expected
next
' Close clipbrd.exe
WshShell.AppActivate("ClipBook Viewer")
WshShell.SendKeys "%F"
WScript.Sleep 1000
WshShell.SendKeys "x"
Sub OpenClipFile(filename)
WshShell.AppActivate("ClipBook Viewer")
WshShell.SendKeys "%W" ' ALT-W for Windows menu
WScript.Sleep 500
WshShell.SendKeys "1" ' Activate Clipboard window
WScript.Sleep 500
WshShell.SendKeys "%F" ' ALT-F for File menu
WScript.Sleep 1000
WshShell.SendKeys "O"
WScript.Sleep 1000
WshShell.SendKeys filenam9e
WScript.Sleep 1000
WshShell.SendKeys "{ENTER}"
WScript.Sleep 1000 ' Wait for "Clear clipboard (yes/no)?"
WshShell.SendKeys "{ENTER}"
End Sub
I'm sure a VBscript hacker could tidy this up considerably and use it
to form a complete test suite. However, while this approach finally gives
us some degree of automation, it is still lacking in several ways:
- The format for the
*.CLP
file is undocumented, so
we cannot add clipboard data of our own, unless we first
copy it to the clipboard, then save it from there using
ClipBook Viewer.
- Automation via sending keys is a very brittle approach.
For instance, the above code was written for the English version
of
clipbrd.exe
. The German or French or Lithuanian versions
of clipbrd.exe
might have completely different keyboard
shortcuts.
- I shudder when looking at those magic delay
time values which the code is ridden with - what if we
run on a really slow system? On a system which has even
more networking problems than the one which I tested the
code with?
- Any process (or user) stealing the window focus while the test is running will break the test.
Hence, next time: Look Ma, no
SendKeys!